CommandBars provides a lot of standard controls for CommandBars -
buttons, popups, edit, combobox, scrollbar, slider, but we always can create
own controls derived from one of them.
This article describes how to create control derived from button
and add some functionality for it.
Let’s create CommandBars button that show active palette color.
1. Create new project using ToolkitPro Appwizard with MenuBar and
Toolbars.
2. Create new class derived from CXTPControlButton (or
CXTPControlPopup for popup button)
class CColorButton : public
CXTPControlButton
{
public:
CColorButton(void);
~CColorButton(void);
};
We need allow our button to save/restore self so need to add
DECLARE_XTP_CONTROL(CColorButton),
IMPLEMENT_XTP_CONTROL(CColorButton, CXTPControlButton)
macros
3. Add COLORREF m_clr variable that will be used to draw button
content and add Get and Set methods
void SetColor(COLORREF clr) {
if (clr != m_clr) {
m_clr = clr;
RedrawParent();
}
}
COLORREF GetColor() const {
return m_clr;
}
4. Override Draw Method and fill rectangle with m_clr color
virtual void Draw(CDC* pDC) {
GetPaintManager()->DrawRectangle(pDC,
GetRect(), GetSelected(),
GetPressed(),
GetEnabled(), GetChecked(), GetPopuped(),
m_pParent->GetType(),
m_pParent->GetPosition());
CRect rcFill(GetRect());
rcFill.DeflateRect(3, 3);
pDC->FillSolidRect(rcFill,
m_clr);
pDC->Draw3dRect(rcFill,
0, 0);
}
5. Now Connect our new button class with button
of toolbar
ON_XTP_CREATECONTROL()
…
int CMainFrame::OnCreateControl(LPCREATECONTROLSTRUCT lpCreateControl)
{
if (lpCreateControl->nID == ID_VIEW_COLOR)
{
lpCreateControl->pControl = new CColorButton();
return TRUE;
}
return FALSE;
}
6. Add Update and Execute handlers in our View
and we’re done.
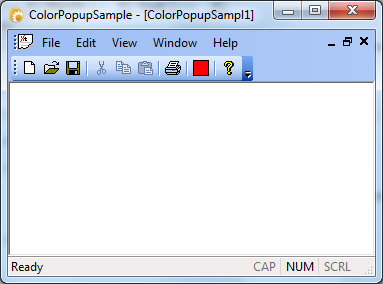
Sample Project: uploads/37/ColorPopupSample.zip - ColorPopupSample.zip
------------- Oleg, Support Team CODEJOCK SOFTWARE SOLUTIONS
|